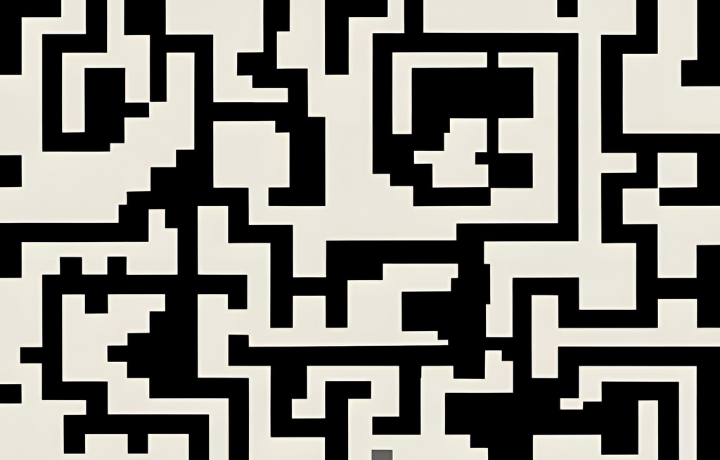
3 Nextjs Design Patterns
3 Nextjs Design Patterns
In this article I want us to learn things that we can't learn in tools like gpteach, I want us to go over common design patterns that software engineers are using when they use nextjs - this will help you understand fundamental parts of nextjs and what makes it good! yes you'll learn it by identifiyng those pattern yourself over timeNext.js Design Pattern: Layout Pattern (Reusing UI Structure Across Pages)
The Layout Pattern in Next.js is used to create a consistent UI structure across multiple pages. It helps avoid code duplication by defining a reusable Layout component that wraps different pages, ensuring a uniform design with shared elements like navigation, headers, footers, and sidebars.
Why Use the Layout Pattern?
1. Reduces Code Duplication - Instead of repeating navigation and footer components on every page, they are defined once in a layout component.2. Improves Maintainability - Changes to shared UI elements need to be updated in a single file instead of multiple pages.
3. Enhances Performance - Components like headers and footers are not reloaded unnecessarily, leading to better performance.
Keeps Pages Focused on Content - Each page only contains the logic and content specific to it, improving readability.
How the Layout Pattern Works in Next.js
1. Create a Layout Component - Define a Layout.tsx (or .jsx) file that includes shared UI elements.2. Wrap Pages with the Layout - Use the Layout component in _app.tsx (for the pages directory) or in the root layout for the app directory in Next.js 14+.
3. Nest Components Inside the Layout - The layout component renders the children prop to display page-specific content while keeping the structure intact.
Where to Use the Layout Pattern?
1. Marketing Websites - Pages like Home, About, and Contact share the same header and footer.2. Dashboards - Admin dashboards with a sidebar and top navigation across all pages.
3. Blogs - Blog posts and category pages sharing the same layout for consistency.
The Layout Pattern in Next.js helps maintain a consistent design, reduces redundancy, and simplifies application structure. It is an essential design pattern for building scalable and maintainable applications, ensuring that shared UI elements are managed efficiently across different pages.
Next.js API Handler Pattern in the App Folder
The API Handler Pattern in Next.js is a structured way to manage API routes inside the app directory. It helps organize API logic, ensuring reusability, maintainability, and consistency across different HTTP methods such as GET, POST, PUT, and DELETE.Why Use the API Handler Pattern?
1. Consistent API Structure – Defines a standardized way to handle different HTTP methods.2. Code Reusability – Allows middleware-like logic for authentication, logging, and error handling.
3. Separation of Concerns – Keeps API logic separate from frontend components, making it easier to manage.
4. Better Maintainability – Reduces redundant code by handling API logic in a single file per resource.
How the API Handler Pattern Works in Next.js (App Folder)
1. Create an API Route – Define an API route inside the app/api/[endpoint]/route.ts file.2. Use HTTP Methods (GET, POST, PUT, DELETE) – Handle different request types inside a single route.ts file.
3. Modularize Common Logic – Use separate functions or middleware-like utilities for authentication, validation, and error handling.
Usage of HTTP Methods in Next.js API Routes
GET (Retrieve Data)1. Used for fetching data from the server.
2. Typically used for public data, user profiles, or product lists.
POST (Create Data)
1.Used for submitting new data to the server.
2. Commonly used for form submissions, user registration, or adding new records.
PUT (Update Data)
1. Used to modify an existing resource.
2. Ideal for updating user details, settings, or order statuses.
DELETE (Remove Data)
1. Used to delete a resource from the database.
2. Commonly used for account deletion, removing posts, or clearing records.
Where to Use the API Handler Pattern?
1. Authentication APIs – Handling user login, registration, and session validation.
2. CRUD Operations – Managing resources like posts, comments, and user profiles.
3. Third-Party Integrations – Fetching data from external APIs, handling webhooks, or processing payments.
The API Handler Pattern in Next.js provides a clean and structured way to manage API routes in the app directory. By organizing different HTTP methods inside a single file and modularizing logic, developers can create efficient, maintainable, and scalable APIs.
Next.js Data Fetching Strategy Pattern (Choosing SSG, SSR, or ISR Wisely)
The Data Fetching Strategy Pattern in Next.js focuses on selecting the most efficient way to retrieve and serve data. Choosing between Static Site Generation (SSG), Server-Side Rendering (SSR), and Incremental Static Regeneration (ISR) impacts performance, scalability, and user experience.Why Use a Data Fetching Strategy?
1. Optimized Performance – Reduces load times by pre-rendering content where possible.2. Efficient Scalability – Minimizes server workload by using static generation where applicable.
3. Better User Experience – Ensures users get the freshest data while balancing speed and accuracy.
4. SEO Benefits – Ensures search engines can index content properly based on the chosen strategy.
Understanding SSG, SSR, and ISR in Next.js
Static Site Generation (SSG) - Best for Pre-Generated Content1. Pages are generated at build time and served statically.
2. Best suited for blogs, documentation, and marketing pages.
3. No server processing is needed per request, making it highly performant.
Server-Side Rendering (SSR) - Best for Real-Time Data
1. Pages are generated on each request using getServerSideProps.
2. Suitable for dynamic content like user dashboards or stock market data.
3. Ensures fresh data but increases server load and response time.
Incremental Static Regeneration (ISR) - Best for Balanced Performance
1. Pages are pre-generated like SSG but can be updated at intervals using revalidate.
2. Useful for product catalogs, news sites, or e-commerce where content changes periodically.
3. Reduces server load while keeping content fresh without requiring full rebuilds.
Where to Use This Pattern?
1. Content-heavy websites – Blogs and articles can use SSG for fast load times.
2. Live data applications – Financial data, sports scores, or stock markets benefit from SSR.
3. E-commerce platforms – ISR allows price updates without regenerating the entire site.
The Data Fetching Strategy Pattern in Next.js ensures that applications load efficiently while serving the right level of freshness for different use cases. Choosing SSG for static content, SSR for real-time data, and ISR for periodic updates leads to better performance, scalability, and user experience.
- Add your email to our mailing list to recevie updates and notifications!
- Stay up to date with AI helping engineers
- Learn faster and join us as a student!